Location
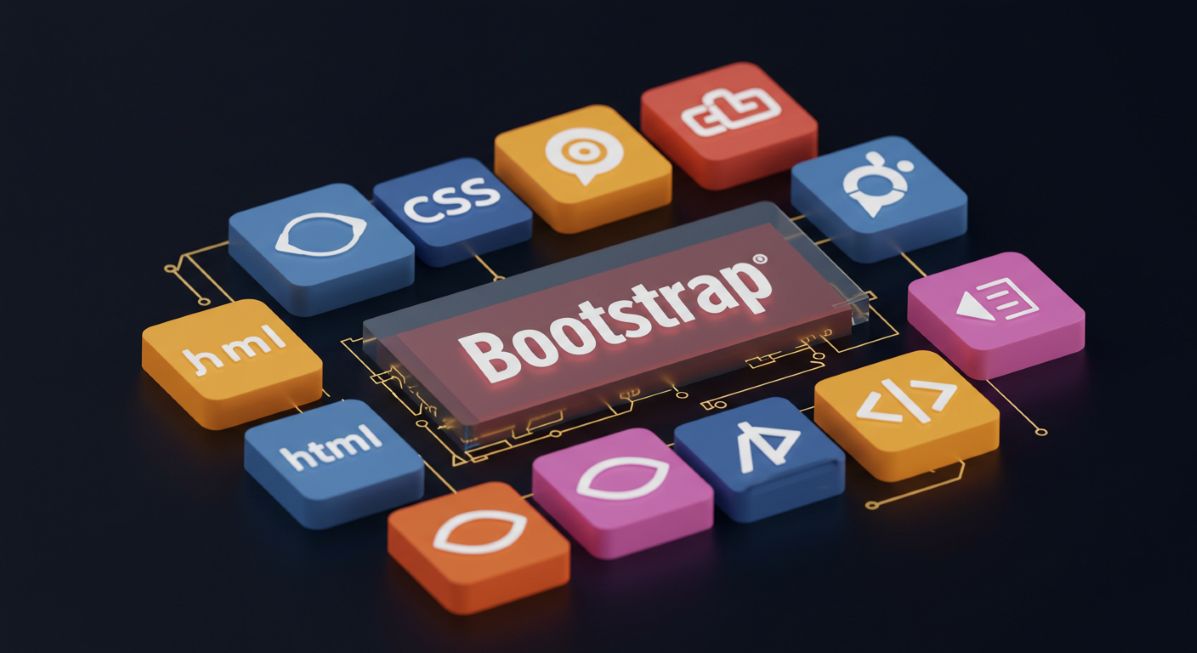
Overview of Bootstrap 5
An overview of Bootstrap 5, its features, and improvements over previous versions. Bootstrap 5 is a popular CSS framework used for designing responsive and modern web interfaces. It provides pre-designed components and utilities that significantly speed up development.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Bootstrap 5 Example</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<h1>Welcome to Bootstrap 5</h1>
</div>
</body>
</html>
Getting Started with Bootstrap 5
Installation Options:
Bootstrap 5 can be added to your project via different methods: using a Content Delivery Network (CDN), downloading the source files, or installing it via npm for integration in build tools.
- Using a CDN (Recommended for beginners):
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.bundle.min.js"></script>
- Using NPM (For advanced workflows):
npm install bootstrap
This method integrates well with module bundlers like Webpack or Parcel.
Understanding Containers in Bootstrap 5
Containers are the fundamental building block of a Bootstrap layout. They are used to wrap site content and provide proper alignment and padding.
Example:
<div class="container">
<p>This is a standard container with fixed width.</p>
</div>
<div class="container-fluid">
<p>This is a full-width container spanning the entire viewport.</p>
</div>
Use container
for a fixed-width container and container-fluid
for a full-width layout.
Basics of the Bootstrap 5 Grid System
The grid system in Bootstrap is a powerful layout tool that uses rows and columns to organize content in a responsive manner.
Example:
<div class="container">
<div class="row">
<div class="col">Column 1</div>
<div class="col">Column 2</div>
<div class="col">Column 3</div>
</div>
</div>
The grid system is based on a 12-column layout. You can customize column spans by specifying the number of columns each item should take up.
Typography Features in Bootstrap 5
Bootstrap includes a comprehensive set of typography styles for headings, paragraphs, lists, and more. These styles ensure that text elements are visually appealing and accessible.
Example:
<h1 class="display-1">Large Display Heading</h1>
<p class="lead">This is a lead paragraph that stands out from regular text.</p>
<p>This is standard paragraph text styled by Bootstrap.</p>
Customizable classes like .display-*
and .lead
make it easy to adjust the size and emphasis of text.
Using Colors in Bootstrap 5
Bootstrap provides predefined classes for text and background colors. These classes follow a consistent naming convention for easy usage.
Example:
<p class="text-primary">This text is styled with the primary color.</p>
<p class="bg-success text-white">This is a success message with a green background and white text.</p>
Color utilities include options for primary, secondary, success, danger, warning, info, light, and dark.
Working with Tables in Bootstrap 5
Bootstrap simplifies table styling with built-in classes for striped rows, borders, and hover effects.
Example:
<table class="table table-striped">
<thead>
<tr>
<th>#</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr>
<td>1</td>
<td>John</td>
<td>28</td>
</tr>
<tr>
<td>2</td>
<td>Jane</td>
<td>32</td>
</tr>
</tbody>
</table>
Additional table classes include .table-bordered
for borders and .table-hover
for hover effects.
Incorporating Images in Bootstrap 5
Bootstrap offers classes for responsive images and additional styling like rounded corners.
Example:
<img src="example.jpg" class="img-fluid rounded" alt="A responsive image with rounded corners">
The .img-fluid
class ensures images scale appropriately within their containers.
Creating Jumbotrons in Bootstrap 5
Jumbotrons highlight important content on your site with a large, attention-grabbing section.
Example:
<div class="p-5 bg-light rounded-3">
<h1>Welcome to Bootstrap 5</h1>
<p>Bootstrap simplifies front-end development with pre-designed components.</p>
</div>
This layout is perfect for call-to-action sections.
Managing Alerts in Bootstrap 5
Alerts are used to display feedback messages to users. Bootstrap provides different contextual classes for alerts.
Example:
<div class="alert alert-warning" role="alert">
Warning! Please check your inputs.
</div>
<div class="alert alert-success" role="alert">
Success! Your operation completed successfully.
</div>
Alerts can be dismissed using the .alert-dismissible
class and a close button.
Buttons and Their Variants in Bootstrap 5
Buttons in Bootstrap come with multiple styling options for different contexts.
Example:
<button type="button" class="btn btn-primary">Primary Button</button>
<button type="button" class="btn btn-outline-secondary">Secondary Outline</button>
Buttons can also be sized using classes like .btn-sm
and .btn-lg
for small and large buttons, respectively.
Grouping Buttons with Bootstrap 5
Button groups allow multiple buttons to be combined into a single element.
Example:
<div class="btn-group">
<button type="button" class="btn btn-secondary">Left</button>
<button type="button" class="btn btn-secondary">Middle</button>
<button type="button" class="btn btn-secondary">Right</button>
</div>
You can also use .btn-toolbar
to group multiple button groups together.
Adding Badges in Bootstrap 5
Badges are used to highlight additional information, such as notifications or item counts.
Example:
<span class="badge bg-primary">New</span>
<span class="badge bg-danger">4</span>
You can combine badges with buttons for interactive elements.
Progress Bars for Visual Feedback in Bootstrap 5
Progress bars indicate task completion status in a visual format.
Example:
<div class="progress">
<div class="progress-bar" role="progressbar" style="width: 50%;">50%</div>
</div>
Additional options include animated progress bars using the .progress-bar-animated
class.
Adding Loading Indicators with Spinners in Bootstrap 5
Spinners visually indicate ongoing processing or loading activities.
Example:
<div class="spinner-border text-primary" role="status">
<span class="visually-hidden">Loading...</span>
</div>
Spinners can also be placed inline with buttons or other elements for better UX.
Visual Examples
Interactive SVG
Click to animate
Progress Tracker
Click to increment
Animated Elements
Continuous animation
Interactive Controls
Animation Settings
More Sections (Summarized):
- BS5 Modals: Interactive dialog boxes with a wide range of options.
- BS5 Tooltip: Add hover-based tooltips to any element using data attributes.
- BS5 Forms: Input fields, validation, and advanced form controls for building user-friendly interfaces.
- BS5 Grid System: Organize and customize layouts with responsive grid options.
This document is a starting point for an in-depth Bootstrap 5 tutorial. Let me know which sections you want fully expanded or additional examples to include!
FAQs
1. What is Bootstrap 5, and how does it differ from previous versions?
Bootstrap 5 is a popular open-source CSS framework used for building responsive and mobile-first web designs. Key differences include:
- Removal of jQuery for better performance.
- Improved grid system with enhanced flexibility.
- New utility classes for faster styling.
- Dark mode support.
- Updated components like cards and buttons.
2. How can I integrate Bootstrap 5 into my project?
Bootstrap 5 can be integrated using:
- CDN: Add the CSS and JavaScript links directly to your HTML file.
- NPM: Install via Node Package Manager for build tool integration.
- Download: Get the source files from the official Bootstrap website.
3. Does Bootstrap 5 support Internet Explorer?
No, Bootstrap 5 has dropped support for Internet Explorer. It focuses on modern browsers that support ES6+ and CSS Grid for better performance and features.
4. What are Bootstrap 5 utility classes, and why are they important?
Utility classes in Bootstrap 5 are pre-defined CSS classes for common styles like margins, paddings, colors, and text alignment. They reduce the need to write custom CSS, speeding up development. Example:
htmlCopy code<div class="p-3 text-center bg-primary text-white">Hello, World!</div>
5. How can I create a responsive layout using Bootstrap 5?
Bootstrap 5’s grid system uses a 12-column layout and supports breakpoints for different screen sizes. Use the col-*
classes to create responsive designs. Example:
htmlCopy code<div class="container">
<div class="row">
<div class="col-md-6">Column 1</div>
<div class="col-md-6">Column 2</div>
</div>
</div>
6. How do I implement dark mode in Bootstrap 5?
Bootstrap 5 includes built-in dark mode support. Use the .bg-dark
and .text-light
classes for dark backgrounds and light text. Alternatively, customize dark mode in your SCSS file. Example:
htmlCopy code<div class="bg-dark text-light p-4">
Dark Mode Content
</div>
7. How do I customize Bootstrap 5 components to match my branding?
Customize Bootstrap using:
- SCSS Variables: Modify default variables to change styles globally.
- Custom CSS: Write your CSS to override default styles.
- Theming: Use Bootstrap’s
@extend
or theme customization features.
8. Can I use Bootstrap 5 with React, Angular, or Vue?
Yes, Bootstrap 5 works seamlessly with modern frameworks:
- Use React-Bootstrap or NG-Bootstrap for framework-specific components.
- Integrate Bootstrap 5 via CDN or npm for global styles and utilities.
9. How do I add animations to Bootstrap 5 components?
Bootstrap 5 doesn’t include built-in animations but works well with libraries like Animate.css or AOS (Animate on Scroll). For interactive components, use JavaScript to trigger animations.
10. What are the best practices for using Bootstrap 5 in large-scale projects?
- Use SCSS for modular styling and better maintainability.
- Keep your design consistent by sticking to utility classes and predefined components.
- Optimize performance by removing unused CSS using tools like PurgeCSS.
- Test responsiveness thoroughly on various devices and screen sizes.